Common Python error types and how to resolve them
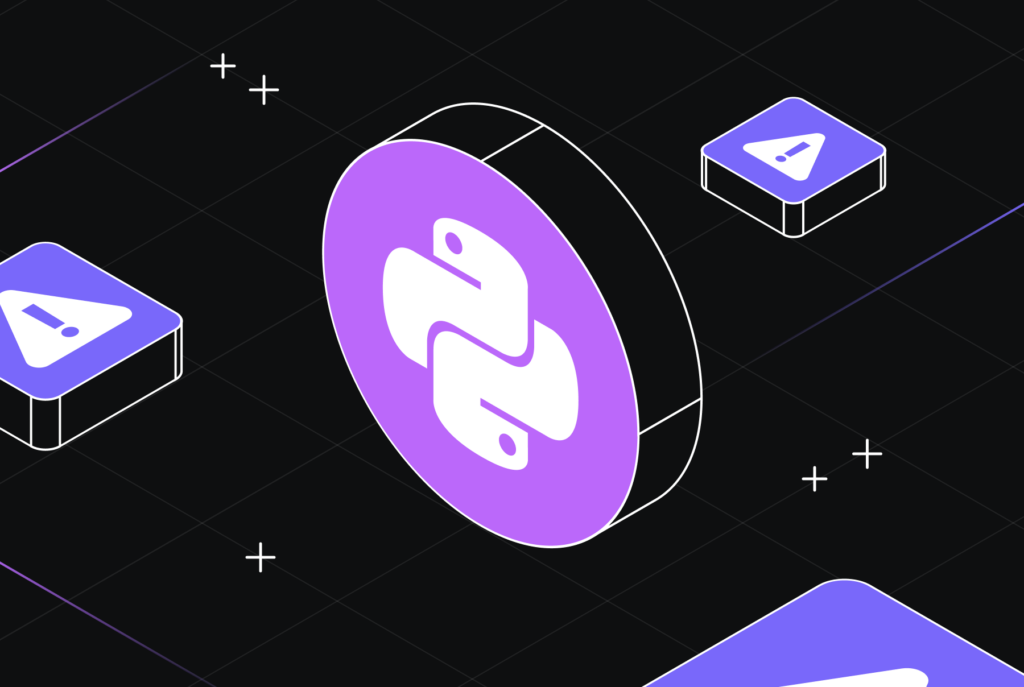
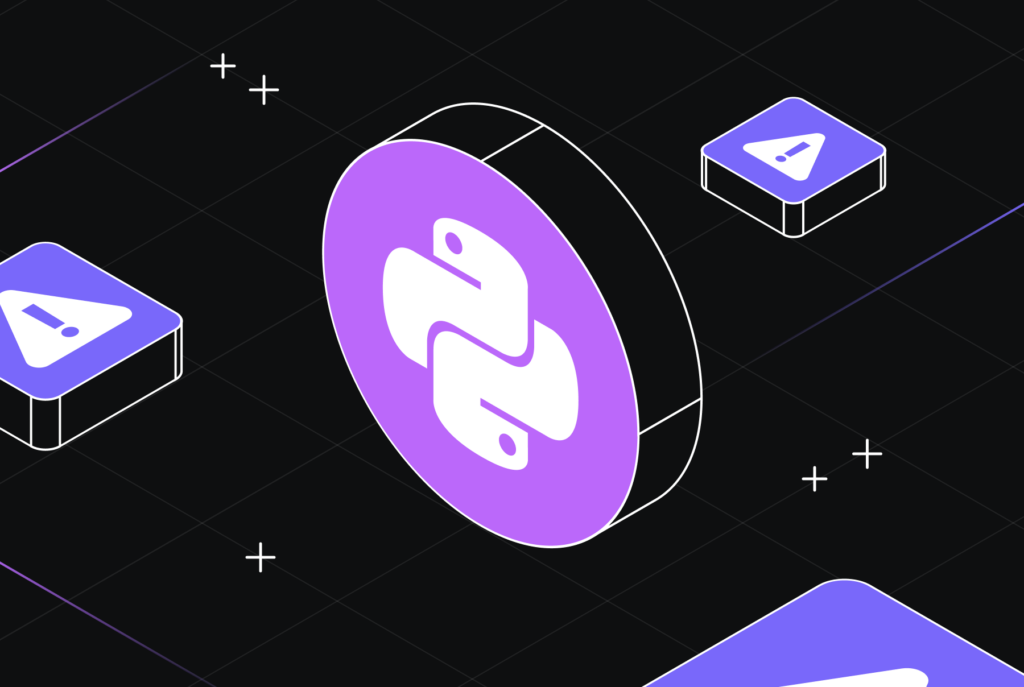
Python errors have a reputation for being cryptic, but they’re actually telling you exactly what went wrong. But whether you’re building a web application, processing data, or creating automation scripts, understanding these errors will remain key for maintaining robust applications.
Python’s exception hierarchy
When dealing with Python errors, it helps to understand how they’re related. Python organizes its exceptions in a hierarchical structure:
BaseException ├── Exception │ ├── ArithmeticError │ │ ├── FloatingPointError │ │ ├── OverflowError │ │ └── ZeroDivisionError │ ├── AttributeError │ ├── ImportError │ │ └── ModuleNotFoundError │ ├── LookupError │ │ ├── IndexError │ │ └── KeyError │ ├── NameError │ │ └── UnboundLocalError │ ├── OSError │ │ ├── FileNotFoundError │ │ ├── ConnectionError │ │ └── PermissionError │ ├── TypeError │ └── ValueError └── SystemExit
Knowing how to interpret and fix these issues efficiently can save hours of debugging time down the road and prevent production issues before they occur. Let’s explore the most common error types you’ll encounter in Python and learn how to handle them with finesse.
SyntaxError
SyntaxError is Python’s way of enforcing its grammar rules. While I recommend you rely on modern IDEs to catch most basic syntax issues, some of these errors can still creep into your codebase, especially when dealing with complex language features or, say, during rapid development cycles.
The most common triggers include:
- missing colons after condition checks or function definitions;
- Wrong indentation in nested blocks;
- Unmatched brackets.
# Wrong: Missing colon after function definition def calculate_average(numbers) return sum(numbers) / len(numbers) # Correct: Proper function definition def calculate_average(numbers): return sum(numbers) / len(numbers)
As a preemptive step, configure your IDE’s linting tools as well as you can and establish consistent code formatting guidelines across your team. Furthermore, if we implement pre-commit hooks and automated code quality checks in our CI/CD pipelines, we can catch most SyntaxErrors before they make it to production.
TypeError
Runtime errors in Python often reveal themselves through type mismatches, particularly in production systems where data flows through multiple transformation stages.
Modern development standards lay stress on type safety through type hints and runtime type checking. And while Python remains dynamically typed, implementing proper type checking can go a long way towards preventing many common TypeError scenarios.
def process_user_data(user_data: dict) -> dict: try: # TypeError if user_data['age'] is a string age_in_months = user_data['age'] * 12 return {'age_months': age_in_months} except TypeError: raise TypeError( f"Age must be a number, got {type(user_data['age']).__name__}" )
ValueError
Now, coming to ValueError situations. This is Python’s way of telling you that while your data type is correct, the actual value isn’t what the code expects. And you’ll see these errors frequently appearing during data processing, input validation, and mathematical operations where values fall outside acceptable ranges.
Implementing robust validation strategies early in your data flow helps catch these issues before they cascade into larger problems. When your code provides clear, actionable error messages, both users and downstream systems know exactly what went wrong and how to fix it.
def validate_user_age(age: int) -> bool: try: if not 0 <= age <= 150: raise ValueError("Age must be between 0 and 150 years") return True except ValueError as e: logger.error(f"Age validation failed: {e}") return False
AttributeError
AttributeError occurs when code attempts to access or modify an attribute or method that isn’t defined for a particular object. If object properties aren’t properly initialized or accessed you can surely expect these errors to surface during runtime.
class UserProfile: def __init__(self, name: str): self.name = name @property def display_name(self): return self.name.title() try: profile = UserProfile(None) print(profile.display_name) # Raises AttributeError except AttributeError: logger.error("Name cannot be None")
To mitigate AttributeErrors, we can implement defensive programming practices using Python’s built-in tools. For instance, the hasattr() function verifies attribute existence before access, while getattr() provides a safe way to access attributes with fallback values. For class-based implementations, we can define all attributes explicitly in the __init__ method, and do consider using dataclasses for more structured management of attributes.
NameError
When a variable or function hasn’t been defined in the current scope, Python raises a NameError. While seemingly straightforward, like some of the other errors above, these errors too can become deceptively complex in larger codebases, especially when dealing with nested scopes and module imports.
def get_user_settings(user_id: int) -> dict: try: settings = load_settings(user_id) # NameError if load_settings isn't defined return settings except NameError: logger.error("Settings loader not properly imported") return {}
You can use static type checkers to catch potential naming issues before runtime, and at the same time fix your IDE configurations to highlight undefined variables as you code. For complex applications, maintaining a clear initialization pattern, be it through dependency injection, configuration objects, or well-defined class attributes can prove to be invaluable in preventing scope-related naming issues.
Production environments require you to stay extra vigilant. Missing imports, undefined variables in conditional blocks, and scope leaks in nested functions are all known to manifest as NameErrors under specific execution paths that might not be immediately obvious during development, so keep an eye.
IndexError
IndexError is one of Python’s runtime errors that manifests when code attempts to access a sequence index that exceeds its bounds. You’ll come across this error type mostly when dealing with list operations, string manipulations, and array processing when the requested index falls outside the valid range viz. [0, n-1], where n represents the sequence length.
Consider the following:
# Common IndexError trigger numbers = [1, 2, 3] print(numbers[4]) # IndexError: list index out of range # Empty list scenario empty_list = [] print(empty_list[0]) # Another IndexError case
To mitigate IndexErrors, it comes highly recommended to implement defensive programming practices. Standard strategies include prevalidating index values against sequence lengths, utilizing Python’s built-in safeguards, and implementing proper error handling:
# Defensive programming approach def safe_access(sequence, index): if index < len(sequence): return sequence[index] return None # Using try-except for graceful handling def process_element(sequence, index): try: return sequence[index] except IndexError: return f"Index {index} exceeds sequence bounds"
For production environments, it’s always a good idea to combine bounds checking with proper logging. Also, keep monitoring to catch potential index violations before they have a detrimental effect on your system’s stability.
TL;DR
Error Type | Common Causes | Solutions |
SyntaxError |
|
|
TypeError |
|
|
ValueError |
|
|
AttributeError |
|
|
NameError |
|
|
IndexError |
|
|
It’s a lazy idea to think error handling is just about catching exceptions. In fact, if you aren’t building resilient applications that can handle unexpected situations gracefully, you’re lagging behind. While in this post we’ve covered the fundamental error types and their prevention strategies, the onus of proactively implementing it lies with you.
The modern Python developer’s toolkit includes powerful debugging aids — from the built-in pdb for command-line debugging to sophisticated IDE debuggers that offer real-time variable inspection and breakpoint management.
But taking it up a notch, modern AI-powered solutions like Qodo can fundamentally transform how developers handle these common errors. Its intelligent code analysis identifies potential issues before runtime, while automatically generating targeted test cases that account for edge cases you might miss.
Imagine working on a web application that processes user data submitted through an API. You write the following function to calculate the length of a user’s phone number to validate it:
def validate_phone_number(phone_number): if len(phone_number) != 10: raise ValueError("Phone number must be exactly 10 digits.") # Example usage phone_number = 1234567890 # Submitted by the user validate_phone_number(phone_number)
Running this code raises a TypeError because len() is being applied to an integer instead of a string.
How Qodo Handles This Error
- Intelligent Code Analysis: During development, Qodo flags the line
if len(phone_number) != 10
as potentially problematic and explains the issue:
“Thelen()
function cannot be applied to an integer. Convert the input to a string first or ensure the input is of the correct type.” - Code generation: Qodo generates a fix with proper type handling to make the function robust, that you can apply directly in your editor.
def validate_phone_number(phone_number): phone_number = str(phone_number) # Ensure input is a string if len(phone_number) != 10: raise ValueError("Phone number must be exactly 10 digits.")
- Generate tests: Qodo automatically generates unit tests to cover happy paths and edge cases, ensuring the method in question covers all bases and there are no unwanted surprises in prod.
All in all, as our idea of software development evolves, the mark of an expert developer isn’t in how well they handle errors — it’s in how rarely they need to. Click here to see Qodo in action.