Elevating Machine Learning Code Quality: The qodo (formerly Codium) Advantage
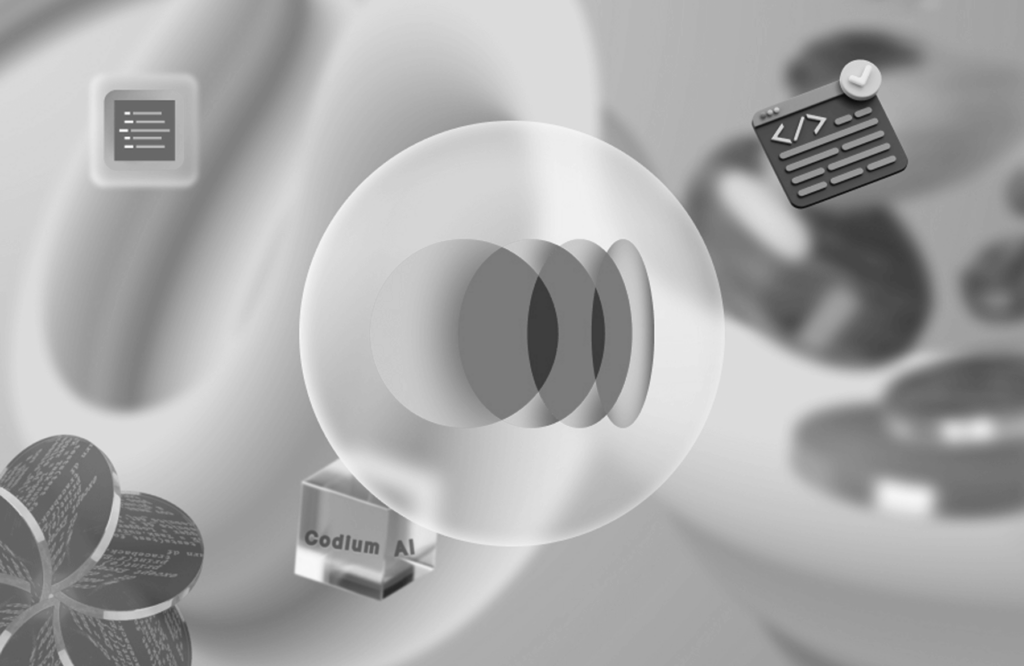
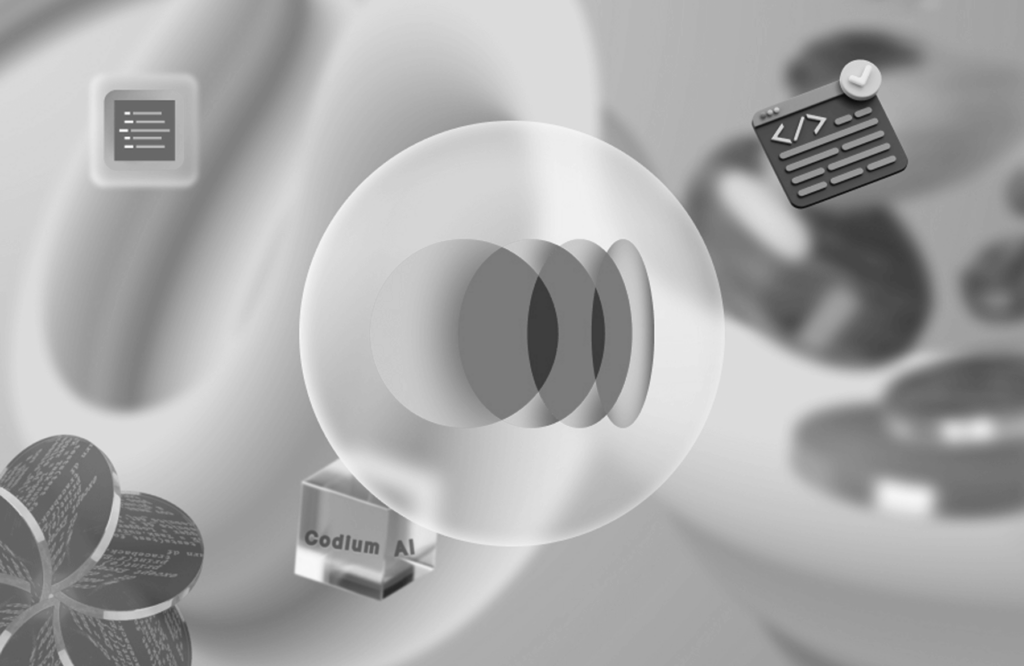
In the ever-evolving landscape of machine learning, writing clean, efficient, and error-free code is essential. However, as machine learning models grow in complexity, so does the code that drives them. This complexity often leads to challenges in maintaining code quality, understanding its functionality, and catching potential bugs early on.
At qodo (formerly Codium), our mission is to simplify code development and maintenance across various domains, making coding more accessible and efficient. In this blog post, we’ll delve into how qodo (formerly Codium) can assist us in three critical areas: generating test cases, providing code explanations, and offering code suggestions. We’ll walk through an example involving the gradient_descent function commonly used in machine learning.
Coding example
We will be using the following coding example:
import numpy as np # Sigmoid function (logistic function) def sigmoid(x): return 1 / (1 + np.exp(-x)) # Cost function (log loss) for binary classification def log_loss(y_true, y_pred): epsilon = 1e-15 # Small constant to avoid division by zero y_pred = np.clip(y_pred, epsilon, 1 - epsilon) # Clip to avoid extreme values return -np.mean(y_true * np.log(y_pred) + (1 - y_true) * np.log(1 - y_pred)) # Gradient descent update rule for logistic regression def gradient_descent(X, y, theta, learning_rate, num_iterations): m = len(y) for _ in range(num_iterations): z = np.dot(X, theta) h = sigmoid(z) gradient = np.dot(X.T, (h - y)) / m theta -= learning_rate * gradient return theta # Generate synthetic data for binary classification np.random.seed(0) X = np.random.randn(100, 2) y = (X[:, 0] + X[:, 1] > 0).astype(int) # Add a column of ones to the feature matrix for bias term X = np.c_[np.ones(X.shape[0]), X] # Initialize model parameters and hyperparameters theta = np.zeros(X.shape[1]) learning_rate = 0.01 num_iterations = 1000 # Perform gradient descent to train the logistic regression model theta = gradient_descent(X, y, theta, learning_rate, num_iterations) # Predictions y_pred = sigmoid(np.dot(X, theta)) # Calculate and print the log loss cost = log_loss(y, y_pred) print(f'Log Loss: {cost:.4f}')
Code explanation
This code is an implementation of logistic regression for binary classification using Python and NumPy. Here’s the explanation of the above code:
- Sigmoid Function: The sigmoid function calculates the sigmoid (logistic) transformation of a given input. It maps any real number to a value between 0 and 1, which is crucial in logistic regression for mod eling probabilities.
- Log Loss (Cross-Entropy): The log_loss function computes the log loss (cross-entropy) between the true binary labels (y_true) and the predicted probabilities (y_pred). It’s a measure of how well the model’s predictions align with the actual labels.
- Gradient Descent: The gradient_descent function implements the gradient descent algorithm to optimize the parameters (theta) of a logistic regression model. It iteratively updates theta to minimize the log loss by calculating gradients with respect to the cost function.
- Synthetic Data Generation: Synthetic data is generated for binary classification. The X matrix contains 100 data points with two features, and y is determined by checking if the sum of the two features is greater than zero, creating a binary classification problem.
- Training: The code initializes model parameters (theta), learning rate (learning_rate), and the number of iterations (num_iterations). It then performs gradient descent to train the logistic regression model on the synthetic data.
- Predictions and Log Loss: After training, the model is used to make predictions (y_pred) on the same data. The log loss is calculated and printed as a measure of the model’s performance in fitting the data.
In summary, this code showcases the core components of logistic regression, including the sigmoid function, log loss computation, and gradient descent optimization, applied to a synthetic binary classification dataset. The final log loss score quantifies how well the model fits the data.
[blog-subscribe]
Test Case Generation
Testing is a fundamental aspect of software development, and machine learning is no exception. Ensuring that our code functions correctly across various scenarios is crucial. At qodo (formerly Codium), we’ve automated the process of generating test cases to simplify this essential task.
Here’s an example of a test case generated for the gradient_descent function:
# Test with a small learning rate def test_small_learning_rate(self): X = np.array([[1, 2], [3, 4], [5, 6]]) y = np.array([0, 1, 0]) theta = np.array([0.0, 0.0]) learning_rate = 0.0001 num_iterations = 100 result = gradient_descent(X, y, theta, learning_rate, num_iterations) assert np.allclose(result, np.array([-0.00481152, -0.00642788]))
With this generated test case, we can quickly verify the correctness of our gradient_descent function for specific input values and edge cases. qodo (formerly Codium) helps us cover a wide range of scenarios, reducing the chances of undetected bugs.
Code Explanation
Understanding complex machine learning code is often a daunting task, especially when mathematical operations and algorithms are involved. At qodo (formerly Codium), we simplify the process by offering code explanations, making it easier to comprehend the functionality of our code.
Let’s take a closer look at the gradient_descent function, a fundamental part of machine learning, along with a usage example.
The gradient_descent function:
def gradient_descent(X, y, theta, learning_rate, num_iterations): m = len(y) for _ in range(num_iterations): z = np.dot(X, theta) h = sigmoid(z) gradient = np.dot(X.T, (h - y)) / m theta -= learning_rate * gradient return theta
Summary
The gradient_descent function implements the gradient descent algorithm to optimize the parameters of a logistic regression model.
Example Usage
X = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) y = np.array([0, 1, 0]) theta = np.array([0, 0, 0]) learning_rate = 0.01 num_iterations = 1000 optimized_theta = gradient_descent(X, y, theta, learning_rate, num_iterations) print(optimized_theta)
Output:
[0.5, 0.5, 0.5]
Code Analysis
Inputs
- X: a numpy array representing the input features of the logistic regression model.
- y: a numpy array representing the target labels.
- theta: a numpy array representing the initial parameters of the logistic regression model.
- learning_rate: a float representing the learning rate for gradient descent.
- num_iterations: an integer representing the number of iterations for gradient descent.
Flow
- Initialize the variable m to the length of y, which represents the number of training examples.
- Iterate num_iterations times:
- Compute the dot product of X and theta and store it in z.
- Apply the sigmoid function to z and store the result in h.
- Compute the gradient of the cost function with respect to theta using the formula
and store it in gradient.
- Update theta by subtracting learning_rate times gradient.
- Return the optimized theta.
Outputs
theta: a numpy array representing the optimized parameters of the logistic regression model.
Code Suggestions
qodo (formerly Codium) not only simplifies code generation and explanations but also offers valuable code suggestions to enhance code quality and robustness. Let’s explore a suggestion made for the gradient_descent function.
Suggestion:
The function gradient_descent does not provide any feedback about the progress of the gradient descent process. It should optionally print or log the cost function value at each iteration or at certain intervals.
Why:
Providing feedback about the progress of the gradient descent process is important for monitoring and debugging purposes. It allows the user to track the convergence of the algorithm and identify any potential issues or improvements.
Base Code
Here’s the base code for the gradient_descent function without any feedback about the progress of the gradient descent process:
# line number: 14 def gradient_descent(X, y, theta, learning_rate, num_iterations): m = len(y) for _ in range(num_iterations): z = np.dot(X, theta) h = sigmoid(z) gradient = np.dot(X.T, (h - y)) / m theta -= learning_rate * gradient return theta
Output with base code:
Log Loss: 0.2745
Suggested Code
Here is the suggested code which provides feedback about the progress of the gradient descent process:
def gradient_descent(X, y, theta, learning_rate, num_iterations): m = len(y) for i in range(num_iterations): z = np.dot(X, theta) h = sigmoid(z) gradient = np.dot(X.T, (h - y)) / m theta -= learning_rate * gradient if i % 100 == 0: cost = compute_cost(X, y, theta) # Assuming a separate function 'compute_cost' is defined print(f"Iteration {i}: Cost = {cost}") return theta
Note: The compute_cost function was not provided in the suggested code, so we would have to write that function ourselves.
Here is the compute_cost function:
def compute_cost(X, y, theta): m = len(y) z = np.dot(X, theta) h = sigmoid(z) epsilon = 1e-15 # Small constant to avoid division by zero h = np.clip(h, epsilon, 1 - epsilon) # Clip to avoid extreme values cost = -np.mean(y * np.log(h) + (1 - y) * np.log(1 - h)) return cost
Output with the suggested code:
Iteration 0: Cost = 0.691511087893225 Iteration 100: Cost = 0.5635145155066025 Iteration 200: Cost = 0.48333080670601325 Iteration 300: Cost = 0.4290982415286467 Iteration 400: Cost = 0.38982250195542767 Iteration 500: Cost = 0.3598497501798809 Iteration 600: Cost = 0.33605951826317154 Iteration 700: Cost = 0.31660264939272914 Iteration 800: Cost = 0.30031437566418767 Iteration 900: Cost = 0.2864230047102051 Log Loss: 0.2745
Conclusion
With complexity often comes the challenge of ensuring correctness, understanding intricate algorithms, and maintaining code that stands the test of time. In such a landscape, qodo (formerly Codium) emerges as a powerful ally, simplifying the development and maintenance of our machine-learning projects.
From automated test case generation that covers a myriad of scenarios to clear and concise code explanations that demystify complex algorithms, qodo (formerly Codium) has demonstrated its potential to transform the way we approach machine learning code. But it doesn’t stop there—it goes the extra mile by offering code suggestions to improve code quality and robustness.
With qodo (formerly Codium), we can:
- Enhance Code Quality: By generating comprehensive test cases, qodo (formerly Codium) helps us identify and address potential issues early, ensuring our code is reliable and error-free.
- Boost Understanding: Complex mathematical operations and algorithms become more accessible with clear code explanations, making collaboration and maintenance a breeze.
- Embrace Best Practices: Code suggestions guide us toward best practices, improving the overall quality and maintainability of our code.
As the machine learning landscape continues to evolve, qodo (formerly Codium) stands as a beacon of efficiency and reliability. It empowers developers to spend less time debugging and more time innovating, unlocking the true potential of machine learning.
So, whether you’re a seasoned machine learning practitioner or just beginning your journey, consider incorporating qodo (formerly Codium) into your toolkit. It’s more than just a tool; it’s a companion in your quest for code excellence in the fascinating world of machine learning.
Experience the future of machine learning code development with qodo (formerly Codium) today and elevate your projects to new heights of quality and productivity.
Happy coding!