Java unit testing: How to use tests as a debugging tool for logic errors
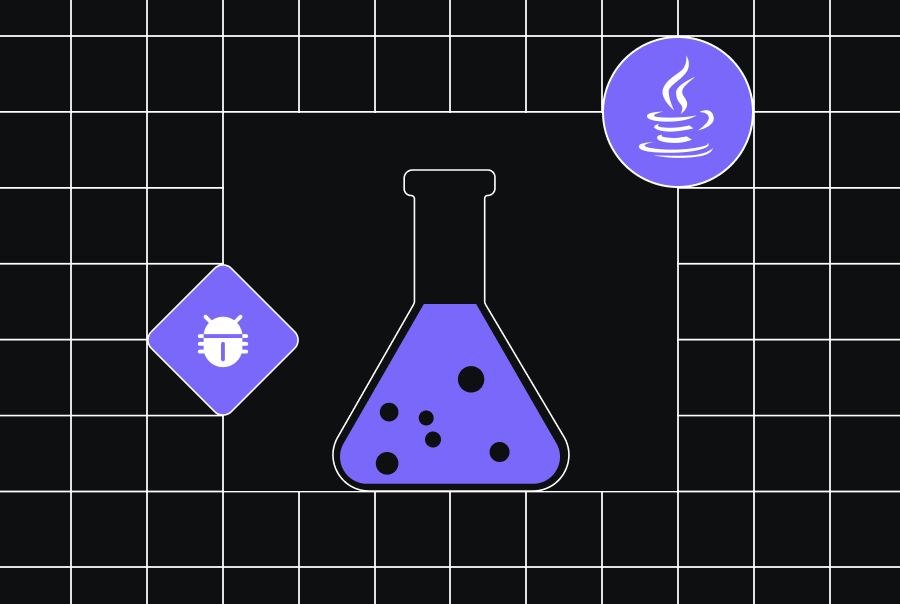
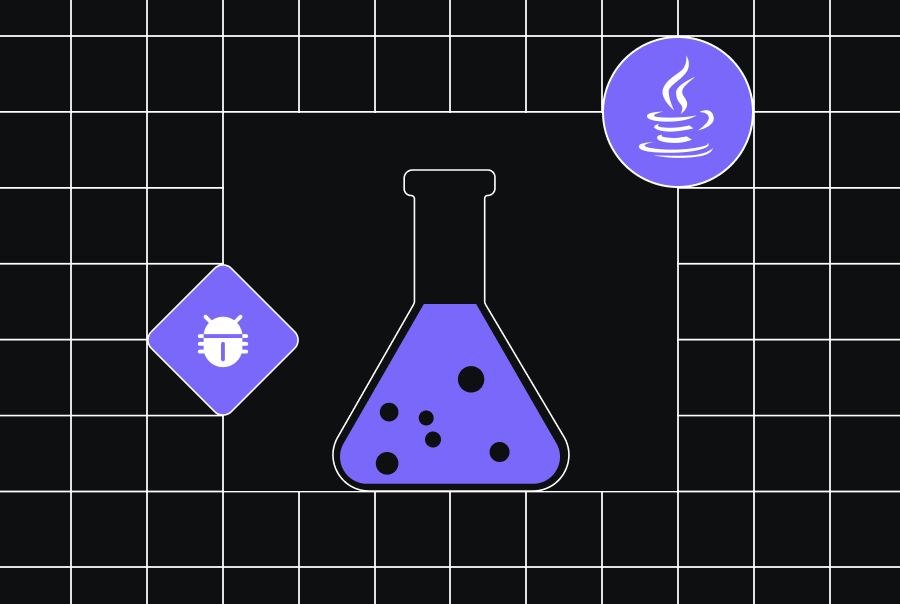
In Java development, logic errors constitute a unique class of defects where code executes flawlessly according to its written instructions while systematically violating business requirements. The disconnect arises when programmatic operations mathematically diverge from domain-specific rules. Think of a tax calculation that subtracts instead of adds deductions, or a scheduling algorithm that ignores daylight saving boundaries. Traditional debugging techniques often prove inadequate for these conceptual mismatches, necessitating a paradigm where test cases become verification protocols for operational semantics.
The peculiar nature of logic errors
Logic errors manifest from a fundamental disconnect — the gap between what you thought you told the computer to do and what you actually instructed it to do. Your code runs, but it’s running the wrong race.
Consider this deceivingly simple calculation method where the intended purpose is to return the final price after applying a percentage reduction:
public double calculateDiscount(double price, double discountPercentage) { return price * discountPercentage; // Logic error! }
The method compiles flawlessly, but there’s a subtle flaw. In fact, 2 of them. The developer forgot to divide the percentage by 100 and also forgot to subtract it from 100, meaning a 20% discount on a $100 item returns $2000 instead of $80 (the discounted price). These errors thrive because they operate within valid syntax while silently corrupting your application’s behavior.
Common logic errors in Java include:
- Off-by-one errors: Iterating one time too many or too few in loops
- Order-of-operations mistakes: Forgetting that `&&` has higher precedence than `||`
- Type confusion: Misunderstanding how different numeric types behave during conversion
- Boundary condition oversights: Failing to handle edge cases at the extremes of your input range
// Flawed loop condition skips final array element for(int i=0; i < transactions.size() - 1; i++) { process(transactions.get(i)); }
The above code compiles successfully but systematically excludes the last transaction which is a classic off-by-one error that unit tests can detect through completeness verification. Interestingly, what makes these errors particularly treacherous is their contextual nature. They often only surface under specific conditions, making them difficult to reproduce and diagnose through conventional debugging.
Test-driven fault isolation
While print statements offer limited runtime inspection, structured unit testing provides systematic fault localization. Consider this enhanced discount test:
@Test public void validateDiscountSemantics() { double baseline = 100.00; double[] discounts = {0.0, 50.0, 100.0, 150.0}; for(double discount : discounts) { double actual = calculator.calculateDiscount(baseline, discount); String msg = String.format("Failed at %.1f%% discount", discount); if(discount <= 100.0) { double expected = baseline * (1 - discount/100); assertEquals(msg, expected, actual, 0.001); } else { // Verify proper error handling assertEquals(msg, 0.00, actual, 0.001); } } }
This test immediately highlights the logic error. The failure message tells you exactly what went wrong: expected 80.00 but got 20.00. It’s not just flagging the issue; it’s providing context for the debugging journey ahead.
But tests can do more than simply identify problems — they can help isolate and understand them. The best debugging tests follow what I call the “GPS principle”: they don’t just tell you something’s wrong; they show you precisely where you took a wrong turn and suggest the correct route.
Techniques for debugging through testing
General advice: create test cases that systematically vary one input parameter while holding others constant. This exposes unexpected interactions between variables that may trigger edge-case failures. But when you’re tracking down a particularly elusive logic error, the following test-driven debugging techniques can be your guide:
1. The hypothesis test
When you suspect a logic error in a specific function, write tests that probe your hypothesis about what’s going wrong:
@Test public void testDiscountHandlesPercentageCorrectly() { DiscountService service = new DiscountService(); // Test with 100% discount to check percentage handling double result = service.calculateDiscount(50.00, 100.0); // If percentage is handled correctly, 100% discount should make item free assertEquals(0.00, result, 0.001); // Test fails: expected 0.00 but was 5000.00 }
This test exposes not just that the function is wrong, but specifically how it’s wrong. And by testing at the boundary ( i.e., 100% discount), we’ve uncovered that the percentage isn’t being converted properly.
2. State progression tests
Stateful components require temporal verification of object state transitions:
@Test public void trackCartStateEvolution() { ShoppingCart cart = new ShoppingCart(); // Phase 1: Initial state assertEquals(0, cart.getItemCount()); // Phase 2: Post-addition cart.addItem(new Item("Monitor", 299.99)); assertEquals(1, cart.getItemCount()); assertEquals(299.99, cart.getSubtotal(), 0.001); // Phase 3: Post-discount cart.applyDiscount(10.0); assertEquals(269.99, cart.getTotal(), 0.001); // 10% of 299.99 }
By tracking the shopping cart’s state through each operation, we can pinpoint exactly where things went wrong — in this case, the discount calculation.
3. Regression test debugging
When fixing a bug, write a test that reproduces the error condition first:
@Test public void testFreeShippingEligibilityEdgeCase() { OrderService service = new OrderService(); Order order = new Order(99.99); // Just below threshold assertFalse(service.isEligibleForFreeShipping(order)); order.updateTotal(100.00); // Exactly at threshold assertTrue(service.isEligibleForFreeShipping(order)); // Test fails: expected true but was false }
This regression test exposes a boundary condition logic error where orders exactly at the $100 threshold aren’t receiving free shipping.
Integrating testing and debugging workflows
Modern IDEs like IntelliJ IDEA and Eclipse create powerful synergies between unit tests and debuggers. You can:
- Set conditional breakpoints inside tests to pause execution only when certain conditions are met
- Use test failure points to jump directly to the problematic code
- Step through test execution to watch your application’s behavior in slow motion
Realize that the goal isn’t just to ensure the tests pass, but to understand why they failed in the first place.
From test failures to code insights
The true power of test-driven debugging lies in transforming test failures into actionable insights about your code’s behavior. When a test fails, it’s telling a story about your logic, so listen carefully.
The fixed version of our discount method reveals the solution:
public double calculateDiscount(double price, double discountPercentage) { return price * (1 - (discountPercentage / 100)); }
The test failure didn’t just highlight the bug; it led us to a more precise understanding of the business logic.
Designing tests with debugging in mind
As you advance in your testing journey, you’ll start writing tests specifically designed to expose subtle logic errors:
- Boundary tests that check behavior at the edges of valid input ranges
- Exhaustive pattern tests that verify behavior across a spectrum of inputs
- Combination tests that expose interactions between different features
Using AI for Java unit tests
While mastering unit tests as debugging tools takes practice, AI-powered solutions like Qodo can significantly accelerate this journey. Qodo’s contextual understanding of your Java codebase helps it automatically generate tests that target potential logic vulnerabilities. Test generation doesn’t just aim for coverage; it’s designed to probe the edge cases where logic errors typically hide.
Well-constructed unit tests serve dual purposes: validating functional requirements and providing forensic evidence for defect analysis. By treating test failures as diagnostic signals rather than mere pass/fail indicators, developers gain deeper insight into system behavior. This approach transforms debugging from reactive error correction to proactive quality assurance.