Synchronous vs. Asynchronous Programming in JavaScript: Key Differences, Examples, and Best Practices
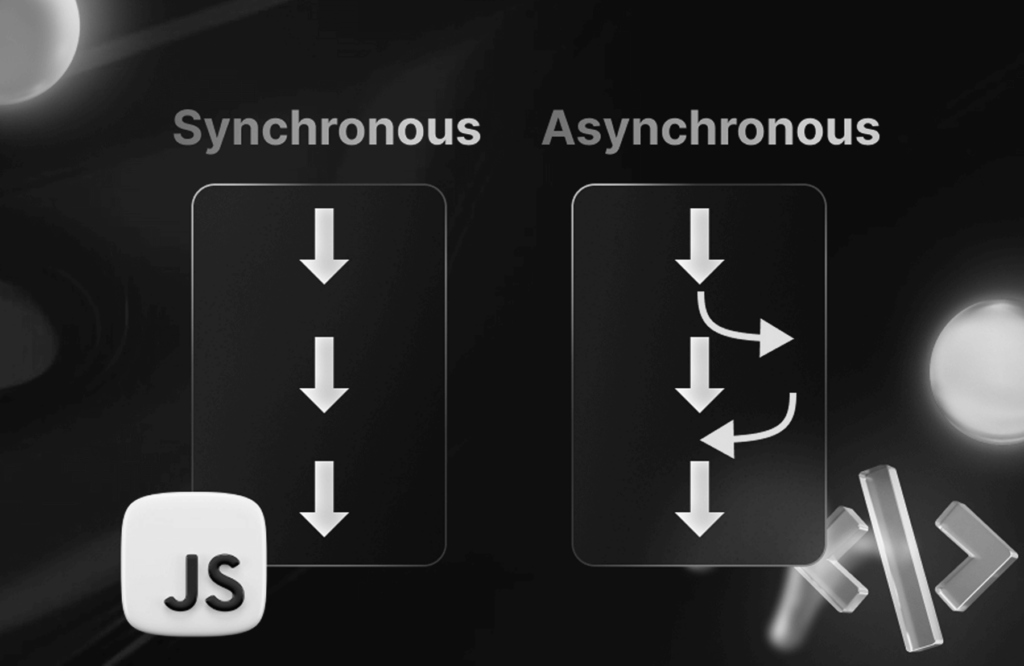
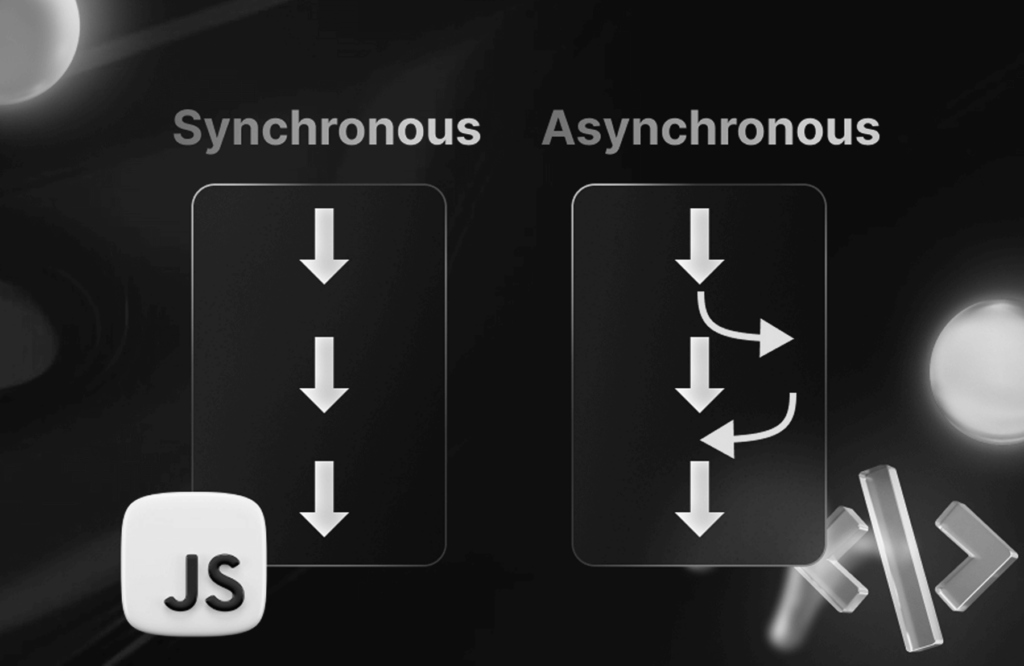
Understanding the benefits and suitability of synchronous vs. asynchronous programming can be extremely helpful for developers aiming to build efficient modern applications.
In this blog, we’ll analyze synchronous and asynchronous programming approaches. We’ll take a deep dive into their main differences, provide several examples of each implementation in JavaScript, examine respective use cases, and explore best practices for each.
Table of Contents
- Understanding Synchronous Programming
- Understanding Asynchronous Programming
- Asynchronous Programming Methods in JavaScript
- Synchronous vs. Asynchronous Programming
- Best Practices for Synchronous and Asynchronous Programming
- Key Points
Understanding Synchronous Programming
When writing JavaScript, code execution follows a sequential order, processing commands one at a time. This is by design, as creating and managing multiple threads can be relatively expensive. Each operation waits for the previous one to complete before proceeding, and the code runs linearly and is easy to follow.
Key Features of Synchronous Programming
- Sequential and single-threaded execution: Operations are performed one after another.
- Blocking behavior: Each task blocks the execution of subsequent tasks until it is completed.
- Predictable flow: The code runs in a linear and understandable manner.
An example of a synchronous code block is below. Each line is processed one by one from top to bottom:
const color = "purple"; const exampleStatement = `My favorite color is ${color}!`; console.log(exampleStatement);
Although synchronous code is usually easy to understand, it has a significant drawback. When programs process synchronous operations that take a long time, they can’t do anything else, making them unresponsive to other events.
Understanding Asynchronous Programming
Asynchronous programming enables developers to overcome the blocking nature of time-consuming operations by scheduling tasks in the background while the main thread processes other commands. This method is standard for handling time-consuming tasks like network requests or file operations.
Key Features of Asynchronous Programming
- Non-blocking behavior: Tasks can run independently of the main program flow.
- Improved performance: Multiple operations can be processed concurrently, enhancing responsiveness. The application remains interactive while performing long-running tasks.
- More complex program flow: The price for concurrency is additional complexity and difficulty in reasoning compared to the sequential nature of synchronous programming.
Asynchronous Programming Methods in JavaScript
When writing Javascript, users have several ways to develop asynchronously.
Callbacks
Callbacks are functions passed as arguments to other functions. Callbacks are used in asynchronous functions when one function must wait for another.
Let’s look at an example below. The `setTimeout()` function specifies a function to be executed as a callback after the timeout period has ended.
function myFunction() { console.log("This will be executed after 5 seconds"); } setTimeout(myFunction, 5000); console.log("This will be executed before the previous log!");
In this example, although the function `myFunction` is mentioned first within the `setTimeout` arguments, it will be executed only after the timeout period.
Although callbacks are easy to set up, they can create nested structures that are difficult to read, understand, and debug. Therefore, callbacks are not typically preferred in modern asynchronous API implementations.
Promises
A promise in JavaScript is an object that represents the eventual completion or failure of an asynchronous operation. It serves as a proxy for a value that may not be known immediately when the promise is created. The promise object allows the program to handle the operation’s eventual success or failure. Promises typically offer a cleaner way to manage asynchronous operations.
A promise’s object state can be pending, fulfilled, or “rejected. When a promise is pending, the result remains undefined as the operation is still running. When a promise is fulfilled, the result contains a value. Finally, if a Promise is rejected, the result is an error object that our program should handle accordingly.
Let’s take a look at one example with a promise:
// Simulate an asynchronous operation function fetchDataFromSomewhere() { return new Promise((resolve, reject) => { console.log("Calling endpoint to fetch data"); const success = true; // simulate success if (success) { resolve(); // if data retrieved successfully } else { reject(); // if failed to fetch data } }); } // Using the Promise fetchDataFromSomewhere() .then((result) => { console.log(result); // success case }) .catch((error) => { console.error(error); // failure case });
In the example above, the function `fetchDataFromSomewhere` defines a promise and attempts to fetch data from a remote location. Our program then uses this promise and handles cases of successful data retrieval or failure to fetch the data.
Async/Await
Async and await simplify working with asynchronous programming and promises. The `async` keyword declares an asynchronous function, which always returns a promise, either explicitly or implicitly.
The `await` keyword can only be used inside asynchronous functions. It pauses the function’s execution until the promise is finalized, allowing asynchronous code to be written more synchronously.
async function myAsyncFunction() { //This is an asynchronous function }
Inside these functions, you can use the `await` keyword before a function that returns a promise to wait until the promise is finalized. This allows writing asynchronous functions that look like synchronous code.
Here’s how the same example we used earlier looks like if we use async/await:
// Simulate an asynchronous operation function fetchDataFromSomewhere() { return new Promise((resolve, reject) => { console.log("Calling endpoint to fetch data"); const success = true; // simulate success if (success) { resolve(); // if data retrieved successfully } else { reject(); // if failed to fetch data } }); } // Using the Promise fetchDataFromSomewhere() .then((result) => { console.log(result); // success case }) .catch((error) => { console.error(error); // failure case }); // Using async/await to handle the Promise async function getData() { try { const result = await fetchDataFromSomewhere(); // Wait until the Promise resolves console.log(result); // Handle success } catch (error) { console.error(error); // Handle error } } // Call the async function getData();
In the example above, the `await fetchDataFromSomewhere()` pauses the execution until the promise resolves or rejects. Typical uses of async/wait are making HTTP requests to APIs, handling file uploads or downloads, database queries, web scraping, real-time applications, and user interfaces.
Synchronous vs. Asynchronous Programming
Aspect | Synchronous Programming | Asynchronous Programming |
Execution order | Sequential and predictable. | Concurrent, but the order is not guaranteed. |
Blocking behavior | Blocks the main program thread. | Does not block the main program thread. |
Complexity | Simple and easy to understand. | Possibly more complex. |
Use Cases | Simple, short tasks require sequential execution. | Network requests, file operations, and long-running processes. |
Choosing Between Synchronous and Asynchronous Programming
When developing with JavaScipt, you usually have to combine both approaches and select the appropriate style when needed. Opt for synchronous code if you need to run tasks in order, and when code simplicity is a priority. Examples include simple scripts with linear flow, utilities, and CPU-intensive tasks that don’t involve significant I/O operations.
Conversely, if your application has network or I/O-heavy operations, such as database queries, network calls, reading from disk, or other long-running computations, you should use asynchronous patterns to keep it responsive.
Best Practices for Synchronous and Asynchronous Programming
Synchronous Programming Best Practices
- Use for simple use cases: This is a good fit for simple and quick computations that don’t require heavy I/O operations.
- Avoid long-running tasks: Synchronous code might not be a great fit for time-consuming operations that could block the main program. This can lead to bad performance and slow user interfaces.
- Keep functions small and focused: Write small, single-purpose functions that perform a unique task. This will improve readability and maintainability.
- Proper error handling: Implement try-catch blocks for effective error management.
- Prefer for CPU-intensive tasks: Synchronous code is suitable for CPU-bound tasks that don’t involve significant I/O operations, such as complex calculations or data processing.
Asynchronous Programming Best Practices
- Promises or async/await over callbacks: Use promises or async/await to handle asynchronous operations since they produce cleaner and more manageable code.
- Proper error handling: Use `.catch` for promises or try-catch blocks with async/await to prevent unhandled promise rejections and maintain application stability.
- Graceful shutdown: When receiving a shutdown signal, gracefully handle asynchronous operations. Log relevant information, cancel or complete outstanding tasks, and close database connections.
- Use promise chaining: When dealing with multiple asynchronous operations that depend on each other, use promise chaining to maintain a transparent and manageable code structure.
- Test asynchronous code: Asynchronous code might be more complex and requires testing frameworks or libraries that support asynchronous flows.
Key Points
In this article, we examined two approaches to Javascript programming: synchronous and asynchronous. We deep-dived into each method’s key characteristics, nuances, and differences. While synchronous programming offers readability and simplicity, its single-threaded nature is unsuitable when parallelism is needed. On the other hand, asynchronous patterns provide the flexibility to handle complex, time-consuming operations in the background without compromising user experience.
It’s important that developers understand the use cases and benefits of each programming style and consider the tradeoffs between simplicity and concurrency when considering the two approaches. By clearly understanding each approach’s unique strengths and applying the appropriate method, developers can build efficient applications across various scenarios.