The Ultimate Java Code Review Checklist: Best Practices for Clean, High-Quality Code
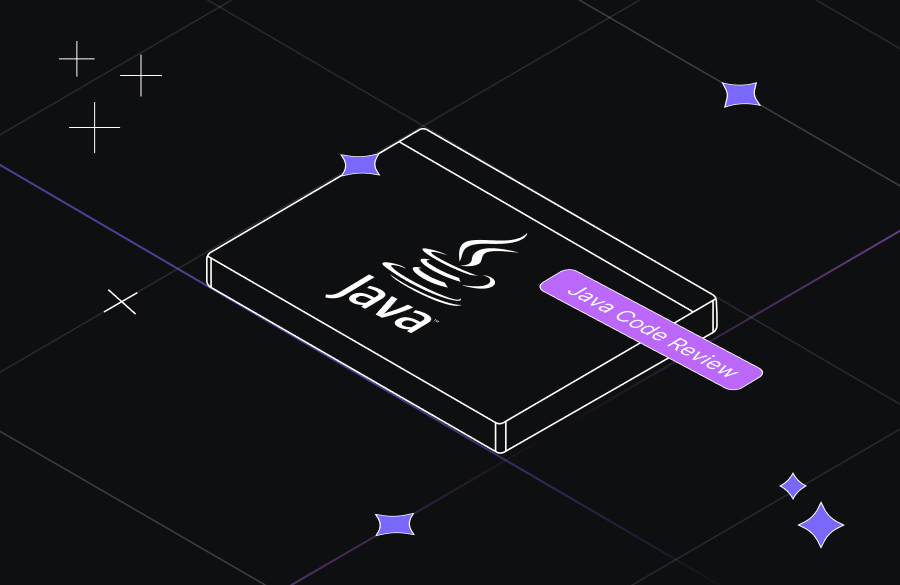
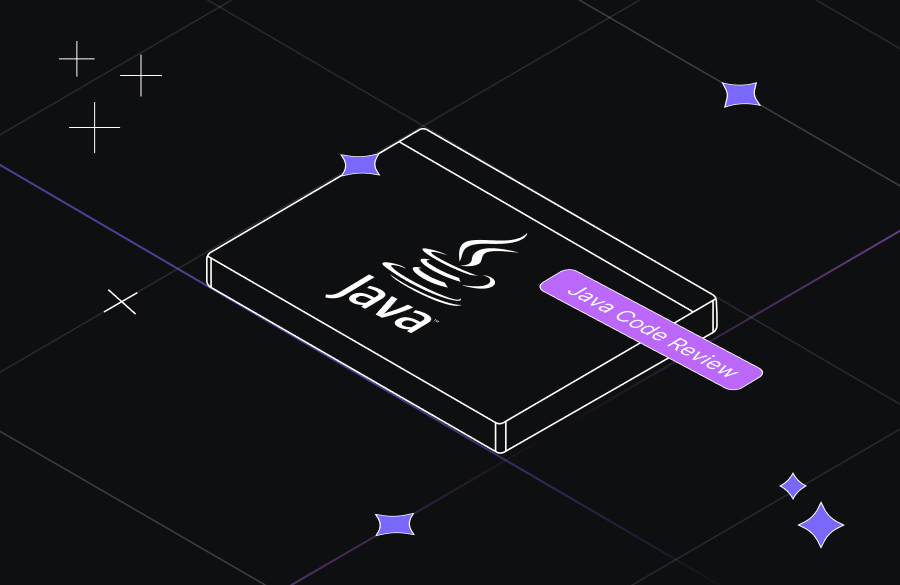
In modern software development, code reviews are a crucial component in delivering high-quality software. They ensure that applications are maintainable, efficient, and bug-free. Without a structured review process, you may face performance issues and introduce bugs and inconsistencies that could put your team at great risk in delivery.
This article will help you understand how important code review is and what you should include in a code review checklist for Java projects.
Role of the Code Review Process
In Java development, maintaining high-quality code is an essential factor in delivering high-quality software. The code review process serves as a quality assurance measurement to discover bugs, improve code structure, and ensure coding standards. It also enhances team collaboration and reduces technical debt, leading to more sustainable source code.
Why Are Code Reviews Crucial for Java Projects?
Java is a highly complex programming language with a strong object-oriented nature designed to enhance a software application’s reliability, scalability, and maintainability, which could be compromised without a rigid code review process. Here are several reasons why code reviews are essential for Java projects.
The strict object-oriented nature of Java requires clear design.
- Java applications enforce object-oriented programming that heavily relies on class hierarchies, inheritance, and encapsulation.
- Poorly structured classes and long inheritance trees or improper methods typically lead to code that is tightly coupled and difficult to maintain.
Large-scale enterprise applications require long-term maintainability.
- Most Java applications are built for large-scale enterprise systems, which require long-term maintainability.
- When code isn’t reviewed, it can become inefficient, bloated, and extremely challenging to refactor over time.
Performance optimization is a key factor in Java.
- To build high-performance systems, memory usage, CPU usage and responsiveness must be optimized. Incorrect implementation of these key factors could cause significant issues such as memory leakages and inefficient loops.
- Code reviews help identify inefficient use of data structures, excessive use of object references, and nested loops.
Exception handling in Java requires mindful reviews.
- Java is a programming language that promotes a strong “checked exception mechanism,” which significantly enhances the reliability of an application. However, it also has a flaw, as it might lead to an excessive use of try-catch blocks without logging them.
- A good code review process not only handles these exceptions well but also uses custom exception classes where necessary and standardized logging mechanisms like SLF4J or Log4J to debug.
Early discovery of security vulnerabilities in Java is essential.
- Java is widely used in security-critical sectors like banking, finance systems, government applications, and e-commerce engines.
- A well-processed code review helps to ensure that applications are secure, use proper encryption hashing, and avoid unsafe Java features like ‘insecure deserialization of objects.’
Java’s multi-threading model can be complex and error-prone.
- Core features, such as concurrent programming and multi-threading models, can introduce deadlocks, synchronization issues, and race conditions if not handled carefully.
- Code reviews can resolve these by correctly using synchronized blocks, deadlocks, and concurrent collections while ensuring thread safety in shared resources.
The Ultimate Java Code Review Checklist
As we now have a clear idea of the importance of code review guidelines, let’s see what should be on a Java code review checklist.
1. Code Readability and Maintainability
- Validate that the code is easy to read and understand.
- Validate whether the variables and methods used adhere to Java naming conventions.
- Ensure comments are clear, relevant, concise, and consistent in formatting and indentation.
// Bad int x = 1; if (x == 1) { System.out.println("Yes"); } // Good int userStatus = 1; if (userStatus == 1) { System.out.println("User is active"); }
2. Adherence to Coding Standards
- Validate that the code aligns with the standard Java conventions like indentation, line length, use of spaces, etc.
- Ensure consistency in the naming and organization of classes, methods, and variables.
// Bad public class myclass{ public void getdata( ){System.out.println("data");}} // Good public class MyClass { public void getData() { System.out.println("data"); } }
3. Error Handling and Exception Management
- Validate that exceptions are well handled with meaningful messages.
- Ensure a clear strategy exists for exception propagation, logging, and recovery.
// Bad try { int result = 10 / 0; } catch (Exception e) { e.printStackTrace(); } // Good try { int result = 10 / 0; } catch (ArithmeticException e) { System.err.println("Cannot divide by zero: " + e.getMessage()); // Log exception properly here }
4. Code Performance, Optimization, and Efficiency
- Ensure that loops, redundant computations, and resource management are implemented efficiently.
- Suggest refinements for code performance without sacrificing readability.
- Look for potential memory leaks or excessive object creation.
// Bad (redundant loop List<String> names = getNames(); for (int i = 0; i < names.size(); i++) { System.out.println(names.get(i)); } // Good List<String> names = getNames(); for (String name : names) { System.out.println(name); }
5. Unit Tests and Test Coverage
- Ensure that tests cover both happy paths and edge cases.
- Validate that unit tests are independent, automated, and run consistently.
- Ensure meaningful assertions are used, and always check if the expected outcome is met.
6. Code Modularity and Reusability
- Ensure the methods/classes used are small and have a single responsibility.
- Ensure that the redundant code has been refactored into reusable methods.
// Bad public void calculateTax() { double tax = salary * 0.18; System.out.println("Tax: " + tax); } // Good public double calculateTax(double salary) { return salary * 0.18; }
- Ensure that design patterns like Factory and Singleton are used where applicable.
7. API and Database Interaction
- Validate that API calls are handled asynchronously.
- Ensure SQL queries are optimized for enhanced performance.
- Check if proper indexing and caching mechanisms are used.
Java Code Review Tools
- SonarQube: One of the most popular tools available in the market for continuous code inspection. Integrates seamlessly with most development environments and automatically analyzes Java code for bugs and vulnerabilities.
- Qodo Gen: An IDE plugin that generates context-aware code and tests, offers smart code suggestions, and helps developers write better tests directly within their environment.
- Qodo Merge: A Git-integrated agent that enhances pull request workflows by reviewing code, highlighting issues, and generating AI-powered suggestions for improvements.
- Checkstyle: An SCA tool designed to validate adherence to Java coding standards. This highly configurable tool can be integrated into your build process to detect style violations automatically.
- PMD: An SCA tool used to identify common programming flaws in Java code, such as unused variables, empty catch blocks, and overly complex methods.
- FindBugs: Another SCA tool focusing on analyzing bytecode to identify potential errors. It also helps detect performance issues and security vulnerabilities.
- JDepend: A tool used to measure the quality of the design of Java code. It calculates metrics related to the structure and dependencies of Java packages and classes.
- JaCoCo: A code coverage tool for Java that measures and generates reports on which parts of the code are covered and not covered by unit tests.
Benefits of Regular Code Reviews
- Fewer bugs in production: Early discovery of bugs helps resolve them earlier than fixing them post-release, which is very costly.
- Enhanced code quality: Scrutinizing the code by multiple developers prevents issues from going unnoticed in production.
- Better team collaboration: Developers learn new techniques, best practices, and solutions from one another.
- Faster development cycle: Discovering and resolving defects or issues helps expedite the delivery cycle as it reduces the complexity of debugging them in a later stage.
- Increased maintainability: Well-reviewed clean code is more modular, reusable, and easier to understand. This leads to better long-term maintainability.
Code Review Pitfalls and How to Avoid Them
1. Rushing Through Code Reviews
Reviewers sometimes rush through code due to time pressure, leading to missed bugs or poor-quality approvals.
How to avoid:
- Set aside time specifically for code reviews.
- Encourage smaller, incremental pull requests to keep reviews manageable.
2. Overlooking Small Issues
While searching for big bugs or design flaws, it’s easy to skip over small but important issues like naming, duplication, or formatting.
How to avoid:
- Minor issues impact maintainability; treat them seriously.
- Use a checklist to consistently catch both big and small problems.
3. Focusing Only on Syntax
Getting stuck on minor formatting or stylistic changes can slow progress and create tension.
How to avoid:
- Prioritize functionality, readability, and logic over nitpicking.
- Use automated tools to handle formatting issues.
4. Ignoring Coding Standards
Without consistent standards, code becomes hard to read and maintain across the team.
How to avoid:
- Follow agreed-upon coding conventions.
- Use linters and formatters to automate standard checks.
5. Not Providing Constructive Feedback
Negative or vague comments like “this is bad” aren’t helpful and can demotivate developers.
How to avoid:
- Give clear, specific suggestions for improvement.
- Focus on the code, not the coder. Keep feedback respectful and helpful.
Final Thoughts
Code reviews in Java projects aren’t just about discovering syntax errors. They help ensure key factors in a project like scalability, performance, security, and maintainability. Since Java is widespread in mission-critical applications across multiple industries, neglecting code review may cause significant impact through costly defects and long-term issues.
FAQs
1. Why is code review critical for Java projects?
Code reviews help catch bugs early, improve code quality, ensure consistency, and promote knowledge sharing across the team. In Java projects, they also help maintain strict type safety and adherence to object-oriented design principles.
2. How do I ensure Java code readability during a review?
Look for clear naming of variables and methods, proper indentation, consistent formatting, and meaningful comments. Encourage short, focused methods and avoid deeply nested logic.
3. How can I enforce Java coding standards in my team?
Use tools like Checkstyle, PMD, or SonarQube to automatically flag violations. Set up shared formatting rules in the IDE and make coding standards part of the review checklist.
4. How do I review Java code for proper unit testing?
Check if tests cover both normal and edge cases. Ensure they are isolated and repeatable and use clear assertions. Also, verify the use of mocking frameworks like Mockito for testing dependencies.